Ajax 방식 요청 처리
- get 방식
BoardController.java
package kr.or.ddit.controller;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import kr.or.ddit.dao.BoardDao;
import kr.or.ddit.vo.BoardVO;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
// 클래스 레벨 요청 경로 지정
@RequestMapping("/board")
public class BoardController {
@RequestMapping(value="/ajaxHome") // 경로
public String ajaxHome() {
log.info("ajaxHome에 왔다");
// forwarding : /views/ajaxHome.jsp
return "ajaxHome"; //jsp 파일 (views 바로 밑에 ajaxHome 파일)
}
}
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
/*
요청URI : /register/hongkd
pathVariable : hongkd
요청방식 : get
*/
$("#rewgisterBtn01").on("click",function(){
$.get("/register/hongkd",function(result){
console.log("result : " + result);
if(result==="SUCCESS") {
alert("SUCCESS");
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
/*
요청URI : /register/hongkd
pathVariable : hongkd
요청방식 : get
동일한 클래스 내에서 같은 이름의 메소드를 사용 : 오버로딩
*/
@ResponseBody
@GetMapping("/register2/{userId}")
public String register01(
@PathVariable("userId") String userId) {
log.info("userId : " + userId);
return "SUCCESS";
}
}
- post 방식
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
/*
요청URI : /register/hongkd
pathVariable : hongkd
요청방식 : get
*/
$("#rewgisterBtn01").on("click",function(){
$.get("/register/hongkd",function(result){
console.log("result : " + result);
if(result==="SUCCESS") {
alert("SUCCESS");
}
});
});
$("#rewgisterBtn02").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
let userObject = {
"userId":userId,
"password":password
};
//아작나써유..(피)씨다타써
/*
요청URI : /register2/hongkd/pw01
pathVariable : hongkd, pw01
요청방식 : post
*/
$.ajax({
url:"/register2/hongkd/pw01",
contentType:"application/json;charset=utf-8",
data:JSON.stringify(userObject),
type:"post",
success:function(result){
console.log("result : ", result);
if(result==="SUCCESS") {
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
/*
요청URI : /register2/hongkd/pw01
pathVariable : hongkd, pw01
요청방식 : post
*/
@ResponseBody
@PostMapping("/register2/{userId}/{password}")
public String register02(
@PathVariable("userId") String userId,
@PathVariable("password") String password) {
log.info("userId : " + userId);
log.info("password : " + password);
return "SUCCESS";
}
}
- post 방식 (파라미터 받아옴)
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#rewgisterBtn03").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//JSON 오브젝트
let userObject = {
"userId":userId,
"password":password
};
//아작나써유..(피)씨다타써
/*
요청URI : /register03
요청파라미터 : {userId:a001,password:java}
요청방식 : post
*/
$.ajax({
url:"/rewgister03",
contentType:"application/json;charset=UTF-8",
data:JSON.stringify(userObject),
type:"post",
success:function(result){
console.log("result : ", result);
if(result==="SUCCESS"){
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
<p><button type="button" id="rewgisterBtn03">경로변수3</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
/*
요청URI : /register03
요청파라미터 : {userId:a001,password:java}
요청방식 : post
*/
@ResponseBody
@PostMapping("/register03")
//비동기로 JSON 데이터가 넘어올 때 @RequestBody를 사용
public String register03(@RequestBody Member member) {
log.info("userId : " + member.getUserId());
log.info("password : " + member.getPassword());
return "SUCCESS";
}
}
JSON오브젝트
- 문자열 매개변수로 받기 => 불가능
=> JSON으로 보냄 -> String으로 받음
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#rewgisterBtn04").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//JSON 오브젝트
let userObject = {
"userId":userId,
"password":password
};
//아작나써유..(피)씨다타써
/*
요청URI : /register04
요청파라미터(JSON->String) : {userId:a001,password:java}
요청방식 : post
*/
$.ajax({
url:"/register04",
contentType:"application/json;charset=UTF-8",
data:JSON.stringify(userObject),
type:"post",
success:function(result){
console.log("result : ", result);
if(result==="FAILED"){
alert("FAILED");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
<p><button type="button" id="rewgisterBtn03">경로변수3</button></p>
<p><button type="button" id="rewgisterBtn04">경로변수4</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
/*
요청URI : /register04
요청파라미터(JSON->String) : {userId:a001,password:java}
요청방식 : post
*/
@ResponseBody
@PostMapping("/register04")
public String register04(String userId,
String password) {
log.info("userId : " + userId);
log.info("password : " + password);
return "FAILED";
}
}
- 문자열 매개변수로 받기 => 가능
=> ajax에서 url의 파라미터로 값 보냄 -> String으로 받음
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#rewgisterBtn05").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//JSON 오브젝트
let userObject = {
"userId":userId,
"password":password
};
//아작나써유..(피)씨다타써
/*
요청URI : /register05
요청파라미터(JSON->String) : {userId:a001,password:java}
요청방식 : post
*/
$.ajax({
url:"/register05?userId="+userObject.userId+"&password="+userObject.password,
contentType:"application/json;charset=UTF-8",
data:JSON.stringify(userObject),
type:"post",
success:function(result){
console.log("result : ", result);
if(result==="SUCCESS"){
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
<p><button type="button" id="rewgisterBtn03">경로변수3</button></p>
<p><button type="button" id="rewgisterBtn04">JSON오브젝트1</button></p>
<p><button type="button" id="rewgisterBtn05">JSON오브젝트2</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
/*
요청URI : /register05
요청파라미터(JSON->String) : {userId:a001,password:java}
요청방식 : post
*/
@ResponseBody
@PostMapping("/register05")
public String register05(String userId,
String password) {
log.info("userId : " + userId);
log.info("password : " + password);
return "SUCCESS";
}
}
+번외 (파라미터를 추가하지 않고 바로 보내는 법)
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#rewgisterBtn0501").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//JSON 오브젝트
let userObject = {
"userId":userId,
"password":password
};
//아작나써유..(피)씨다타써
/*
요청URI : /register0501
요청파라미터(JSON->String) : {userId:a001,password:java}
요청방식 : post
*/
$.ajax({
url:"/register0501",
data:userObject,
type:"post",
success:function(result){
console.log("result : ", result);
if(result==="SUCCESS"){
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
<p><button type="button" id="rewgisterBtn03">경로변수3</button></p>
<p><button type="button" id="rewgisterBtn04">JSON오브젝트1</button></p>
<p><button type="button" id="rewgisterBtn05">JSON오브젝트2</button></p>
<p><button type="button" id="rewgisterBtn0501">JSON오브젝트501</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
/*
요청URI : /register0501
요청파라미터(JSON->String) : {userId:a001,password:java}
요청방식 : post
*/
@ResponseBody
@PostMapping("/register0501")
public String register0501(String userId,
String password) {
log.info("userId : " + userId);
log.info("password : " + password);
return "SUCCESS";
}
}
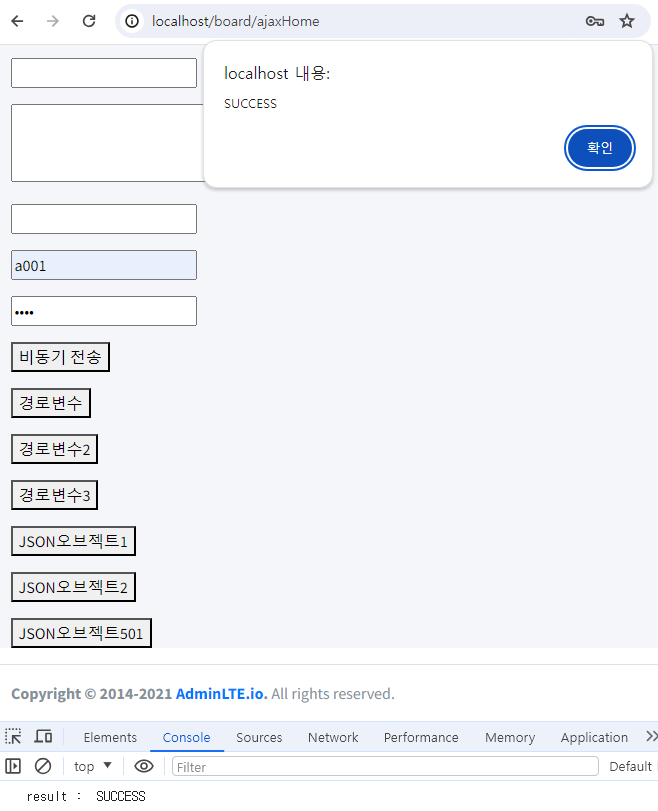
- @PathVariable, @RequestBody 사용 => 가능
@PathVariable과 @RequestBody 모두 한꺼번에 사용할 수 있음
PathVariable : url 파라미터 값
RequestBody : JSON 데이터 값
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#rewgisterBtn06").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//JSON 오브젝트
let userObject = {
"userId":userId,
"password":password
};
$.ajax({
url:"/register06/hongkd",
contentType:"application/json;charset=utf-8",
data:JSON.stringify(userObject),
type:"post",
success:function(result){
console.log("result : " + result);
if(result==="SUCCESS") {
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
<p><button type="button" id="rewgisterBtn03">경로변수3</button></p>
<p><button type="button" id="rewgisterBtn04">JSON오브젝트1</button></p>
<p><button type="button" id="rewgisterBtn05">JSON오브젝트2</button></p>
<p><button type="button" id="rewgisterBtn0501">JSON오브젝트501</button></p>
<p><button type="button" id="rewgisterBtn06">JSON오브젝트6</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
@ResponseBody
@PostMapping("/register06/{userId}")
public String register06(@PathVariable("userId") String userId,
@RequestBody Member member) {
log.info("userId : " + userId);
log.info("userId : " + member.getUserId());
log.info("password : " + member.getPassword());
return "SUCCESS";
}
}
- 객체 배열 타입 (List<Member>)
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#rewgisterBtn07").on("click",function(){
let userObjectArray = [
{"userId":"name01", "password":"pw01"},
{"userId":"name02", "password":"pw02"}
];
$.ajax({
url:"/register07",
contentType:"application/json;charset=UTF-8",
data:JSON.stringify(userObjectArray),
type:"post",
dataType:"text",
success:function(result){
console.log("result : " + result);
if(result==="SUCCESS"){
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="putBtn">비동기 전송</button></p>
<p><button type="button" id="rewgisterBtn01">경로변수</button></p>
<p><button type="button" id="rewgisterBtn02">경로변수2</button></p>
<p><button type="button" id="rewgisterBtn03">경로변수3</button></p>
<p><button type="button" id="rewgisterBtn04">JSON오브젝트1</button></p>
<p><button type="button" id="rewgisterBtn05">JSON오브젝트2</button></p>
<p><button type="button" id="rewgisterBtn0501">JSON오브젝트501</button></p>
<p><button type="button" id="rewgisterBtn06">JSON오브젝트6</button></p>
<p><button type="button" id="rewgisterBtn07">JSON오브젝트7</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
@ResponseBody
@PostMapping("/register07")
public String register07(
@RequestBody List<Member> memberList
) {
for (Member member : memberList) {
log.info("userId : " + member.getUserId());
log.info("password : " + member.getPassword());
}
return "SUCCESS";
}
}
중첩된 자바빈즈
- 중첩된 자바빈즈(1:1)
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#registerBtn08").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//Member 자바빈 클래스 타입
let userObject = {
"userId":userId,
"password":password,
"address":{
"postCode":"12345",
"location":"대전 중구 대흥동 3"
}
};
$.ajax({
url:"/register08",
contentType:"application/json;charset=UTF-8",
data:JSON.stringify(userObject),
type:"post",
dataType:"text",
success:function(result){
console.log("result : " + result);
if(result==="SUCCESS"){
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="registerBtn08">중첩된자바빈즈</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
@ResponseBody
@PostMapping("/register08")
public String register08(
@RequestBody Member member
) {
log.info("member : " + member);
log.info("userId : " + member.getUserId());
log.info("password : " + member.getPassword());
Address address = member.getAddress();
if(address != null) {
log.info("postCode : " + address.getPostCode());
log.info("location : " + address.getLocation());
}
return "SUCCESS";
}
}
- 중첩된 자바빈즈(1:N)
ajaxHome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<script type="text/javascript" src="/resources/js/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#registerBtn08").on("click",function(){
let userId = $("#userId").val();
let password = $("#password").val();
//Member 자바빈 클래스 타입
let userObject = {
"userId":userId,
"password":password,
"address":{
"postCode":"12345",
"location":"대전 중구 대흥동 3"
},
"cardList":[
{"no":"23456", "validMonth":"24/05"},
{"no":"12342", "validMonth":"27/03"}
]
};
$.ajax({
url:"/register08",
contentType:"application/json;charset=UTF-8",
data:JSON.stringify(userObject),
type:"post",
dataType:"text",
success:function(result){
console.log("result : " + result);
if(result==="SUCCESS"){
alert("SUCCESS");
}
}
});
});
});
</script>
<h2>ajaxHome.jsp</h2>
<form>
<p><input type="text" id="boardNo" name="boardNo"></p>
<p><input type="text" id="title" name="title"></p>
<p>
<textarea rows="3" cols="30" id="content"
name="content"></textarea>
</p>
<p><input type="text" id="writer" name="writer"></p>
<p><input type="text" id="userId" name="userId"></p>
<p><input type="password" id="password" name="password"></p>
<p><button type="button" id="registerBtn08">중첩된자바빈즈</button></p>
</form>
MemberController.java
package kr.or.ddit.controller;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import kr.or.ddit.service.MemberService;
import kr.or.ddit.vo.Address;
import kr.or.ddit.vo.Member;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class MemberController {
@ResponseBody
@PostMapping("/register08")
public String register08(
@RequestBody Member member
) {
log.info("member : " + member);
log.info("userId : " + member.getUserId());
log.info("password : " + member.getPassword());
Address address = member.getAddress();
if(address != null) {
log.info("postCode : " + address.getPostCode());
log.info("location : " + address.getLocation());
}
List<Card> cardList = member.getCardList();
if(cardList != null) {
for (Card card : cardList) {
log.info("no : " + card.getNo());
log.info("validMonth : " + card.getValidMonth());
}
}
return "SUCCESS";
}
}
'스프링 프레임워크' 카테고리의 다른 글
[스프링 프레임워크] 20장 모델을 통한 데이터 전달 (0) | 2024.05.09 |
---|---|
[스프링 프레임워크] 19장 파일업로드 Ajax 방식 요청 처리 (0) | 2024.05.08 |
[스프링 프레임워크] 17장 파일 업로드 (0) | 2024.05.03 |
[스프링 프레임워크] 16장 컨트롤러 요청 처리3(폼 필드 요소 값을 매개변수로 처리) (1) | 2024.05.02 |
[스프링 프레임워크] 15장 컨트롤러 요청 처리2(Date 타입 처리, DateTimeFormat 애너테이션) (1) | 2024.05.01 |